I developed a tool to help speed up the Bukkit/Spigot plugin development cycle. It’s quick and easy to use, doesn’t require much set up and here’s how to use it!
WatchdogReloader
WatchdogReloader is a simple utility plugin I made, which reloads a plugin when the .jar file gets changed inside the plugins folder. It monitors the folder using a watchdog service.
You can download the plugin here: https://github.com/WouterGritter/WatchdogReloader#download
You just have to install the plugin, make sure the plugin you want to watch is loaded (by typing /reload once) and typing the command /watch <plugin>. It will tell you how to do this in chat as well!
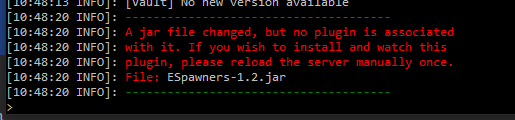
FTP-reachable development server
Now that the plugin gets reloaded if the jar file gets changed, it’s time to speed up the process of getting the jar file in the plugins folder of your development server. Whether you host your development server locally or externally, this will work! You just have to make sure that the server files are reachable through FTP. If you host your server externally this is easy enough to do and you probably already have this set up.
If you are hosting your development server locally on Windows, I recommend downloading this program: https://www.sentex.ca/~mwandel/ftpdmin/
It’s old but gold. To use it for our purposes, put the .exe file in the server folder and write a batch file containing the following:
ftpdmin.exe -ha 127.0.0.1 .
This will set the host address (-ha) to 127.0.0.1, so nobody can reach the server outside of our machine. The root folder of the FTP server will be where the .exe file is placed in (.).
The username and password is not checked when a connection is made. You can enter whatever you’d like.
Maven FTP artifact
Now that you’ve got an FTP server running, it’s time to tell maven to upload the assembled .jar file of your plugin to it. It’s actually fairly simple!
Put this inside your pom.xml file, you don’t have to change anything about it:
<build>
<plugins>
<plugin>
<groupId>org.codehaus.mojo</groupId>
<artifactId>wagon-maven-plugin</artifactId>
<version>1.0</version>
<executions>
<execution>
<id>upload-assembly</id>
<phase>install</phase>
<goals>
<goal>upload-single</goal>
</goals>
<configuration>
<serverId>ftp-repository</serverId>
<fromFile>${project.build.directory}/${project.build.finalName}.jar</fromFile>
<url>${ftpUrl}</url>
<toFile>plugins/${project.build.finalName}.jar</toFile>
</configuration>
</execution>
</executions>
</plugin>
</plugins>
</build>
Once that’s done, you need to put this in your global settings.xml file:
<servers>
<server>
<id>ftp-repository</id>
<username>FTP_USERNAME</username>
<password>FTP_PASSWORD</password>
</server>
</servers>
<profiles>
<profile>
<id>FTP upload</id>
<activation>
<activeByDefault>true</activeByDefault>
</activation>
<properties>
<ftpUrl>ftp://FTP_SERVER/</ftpUrl>
</properties>
</profile>
</profiles>
You have to change the FTP_USERNAME, FTP_PASSWORD and FTP_SERVER values to suite your environment.
Testing your plugin
Now that you are done with the installation process, the process of compiling the plugin, uploading/moving the plugin and reloading the server is greatly reduced in time. It’s just a matter of double clicking the install button and everything will be done for you!
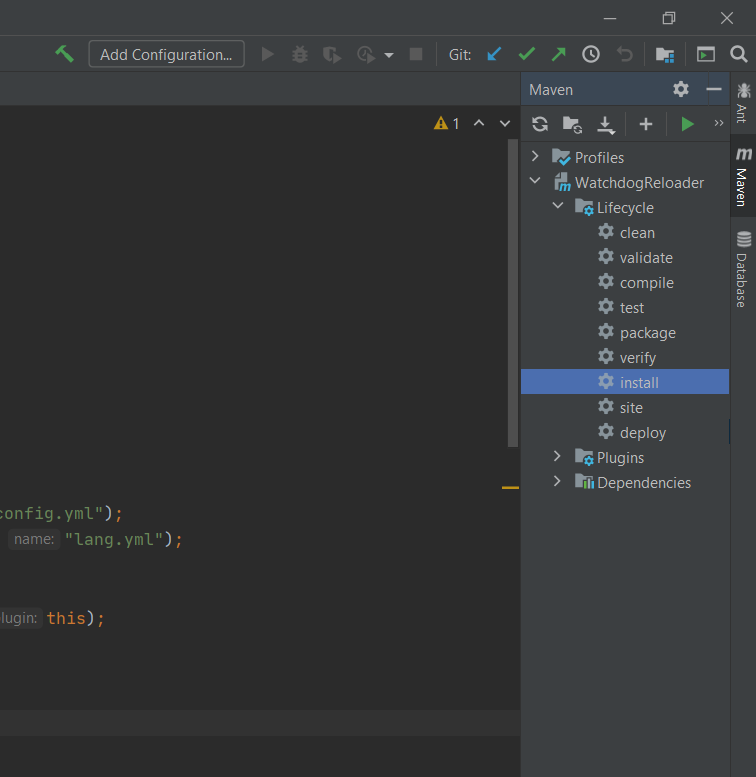
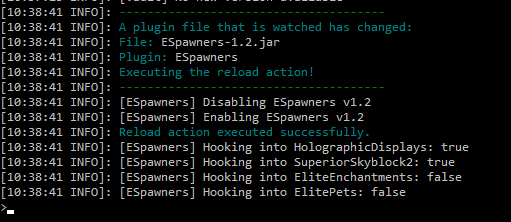